RESTful JSON Web Service - Jersey (v1.19)
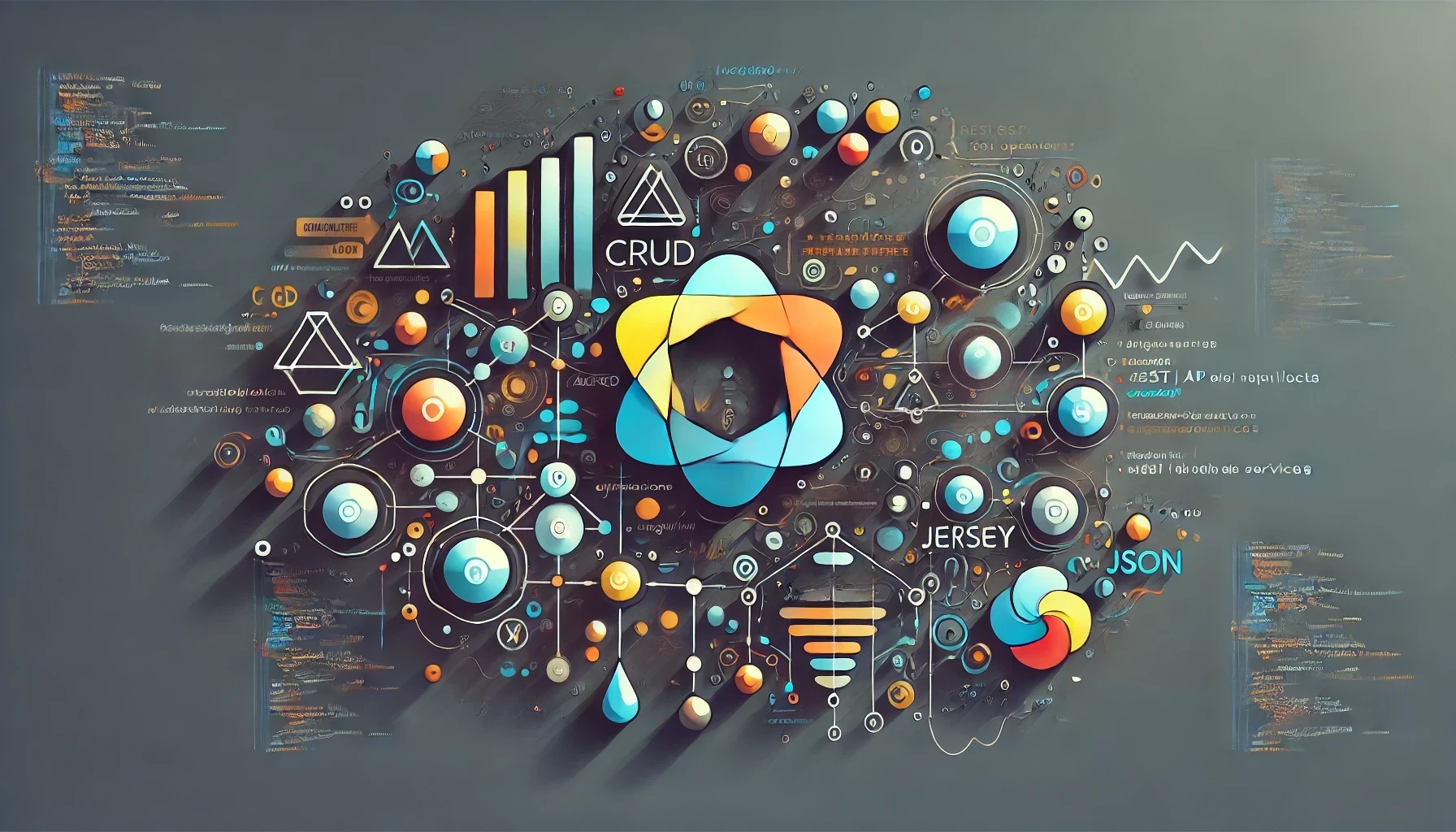
I started out once again looking into AngularJS. This time, the goal was to integrate some CRUD operations using either $http
or $resource
.
AngularJS $http
Using the $http Service in AngularJS to Make AJAX Requests$q
is the angular promise library
AngularJS $resource
Creating a CRUD App in Minutes with Angular's $resource
Most Single Page Applications involve CRUD operations. If you are building CRUD operations using AngularJS, then you can leverage the power of the $resource
service. Built on top of the $http
service, Angular’s $resource
is a factory that lets you interact with RESTful backends easily. So, let’s explore $resource
and use it to implement CRUD operations in Angular.
I read some articles about the pros and cons of both approaches. As I began to implement each approach, I realized I did not have a solid backend to tie into. As a result, I started to pursue using Jersey as a library for creating RESTful web services.
In the past, I have used both Jakarta Struts and Spring to do web services. But, at Explorys, our projects use Jersey. It also seems we have a project or two that use RESTEasy, but I may dig into that another time.
I got a little frustrated setting up the project as the specific goals I wanted to achieve, I could not find a beginning to end example. So, I created a GitHub account and uploaded my project to it! I wanted to use Maven, Jersey, and not have a web.xml
file within the project (annotations only).
https://github.com/DEV3L/RESTfulJSONDev3l
I have some step-by-step instructions that I will paste below, but the result boils down to two RESTful endpoints:
http://services-dev3l.rhcloud.com/RESTfulJSONDev3l/resources/example
http://services-dev3l.rhcloud.com//RESTfulJSONDev3l/resources/example/query
Using this template project, my next step is to create a "standard" set of CRUD operations for a dataset. The first step is to do this against an in-memory static list, but eventually, the services will interact with a database using JOOQ.
RESTfulJSONDev3l
Jersey JSON RESTful web service project (template) - uses annotations without removing the need for a web.xml
- com.sun.jersey : v1.19
Usage Instructions to Pull down and use template project from GitHub
- From command line retrieve project from GitHub
git clone https://github.com/DEV3L/RESTfulJSONDev3l.git
- Run maven package from inside the created directory
mvn clean package
RESTfulJSONDev3l.war
can be deployed to the container of choice- http://services-dev3l.rhcloud.com/RESTfulJSONDev3l/resources/example
- My personal OpenShift server I deploy projects to
- http://services-dev3l.rhcloud.com/RESTfulJSONDev3l/resources/example
- Use Maven to resolve the dependencies and create Eclipse dynamic web project and class/project files
mvn eclipse:eclipse -Dwtpversion=2.0
- Import the project into Eclipse as an existing project
Project Creation Steps:
Assumes Maven already installed
- Create a new Dynamic Web Project using Maven from the console
mvn archetype:create -DgroupId=RESTfulJSONDev3l -DartifactId=RESTfulJSONDev3l -DarchetypeArtifactId=maven-archetype-webapp
- Copy the created project to an Eclipse workspace
/Workspaces/Eclipse/Sandbox/
- Update the
pom.xml
:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>RESTfulJSONDev3l</groupId>
<artifactId>RESTfulJSONDev3l</artifactId>
<packaging>war</packaging>
<version>1.0-SNAPSHOT</version>
<name>RESTfulJSONDev3lApp</name>
<url>http://maven.apache.org</url>
<build>
<finalName>RESTfulJSONDev3l</finalName>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.7</source>
<target>1.7</target>
</configuration>
</plugin>
<plugin>
<!-- Java EE 6 doesn't require web.xml, Maven needs to catch up! -->
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>2.4</version>
<configuration>
<failOnMissingWebXml>false</failOnMissingWebXml>
</configuration>
</plugin>
</plugins>
</build>
<dependencies>
<!-- Jersey REST Dependencies -->
<dependency>
<groupId>asm</groupId>
<artifactId>asm</artifactId>
<version>3.3.1</version>
</dependency>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20140107</version>
</dependency>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-bundle</artifactId>
<version>1.19</version>
</dependency>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-server</artifactId>
<version>1.19</version>
</dependency>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-core</artifactId>
<version>1.19</version>
</dependency>
</dependencies>
</project>
- Use Maven to resolve the dependencies and create Eclipse project files
- From the console within the project
mvn eclipse:eclipse -Dwtpversion=2.0
- From the console within the project
- Import the project into Eclipse as an existing project
- Delete
index.jsp
located atsrc/main/webapp
- Delete
web.xml
located atsrc/main/webapp/WEB-INF
- Create a
src/main/java
source folder- Could use the existing
src/main/resource
that comes with the archetype, but this is not "best practice" Maven
- Could use the existing
- Create a Bean/POJO with an
@XmlRootElement
class attribute- The
@XmlRootElement
tells our application how this can be serialized
- The
package com.dev3l.jersey.bean;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class DataBean {
private String data;
public DataBean() {
this(null);
}
public DataBean(String data) {
this.data = data;
}
public final String getData() {
return data;
}
public final void setData(String data) {
this.data = data;
}
}
- Create an
ApplicationConfig
with an@ApplicationPath
attribute- The
@ApplicationPath
is the root path of the web service calls
- The
package com.dev3l.jersey.config;
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("resources") // set the path to REST web services
public class ApplicationConfig extends Application {}
- Create an Example resource with
@PATH
,@GET
and@Produces(MediaType.APPLICATION_JSON)
package com.dev3l.jersey.resource;
import java.util.ArrayList;
import java.util.List;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.GenericEntity;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import com.dev3l.jersey.bean.DataBean;
@Path("example")
public class ExampleResource {
private static List<DataBean> dataBeans = new ArrayList<DataBean>();
static {
dataBeans.add(new DataBean("first"));
dataBeans.add(new DataBean("second"));
dataBeans.add(new DataBean("third"));
dataBeans.add(new DataBean("fourth"));
}
@GET
@Produces(MediaType.APPLICATION_JSON)
public DataBean getDataBean() {
return new DataBean("test_data_bean");
}
@GET
@Path("query")
@Produces(MediaType.APPLICATION_JSON)
public Response query() {
GenericEntity<List<DataBean>> entity = new GenericEntity<List<DataBean>>( dataBeans ){};
return Response.ok().entity(entity).build();
}
}
- Add the project to a Java web container
- Default Tomcat 7.0
- Start the server
- Access the endpoints via a browser URL
2015040 - I have switched to using RESTEasy instead of Jersey. They are very similar and aside from a few differently named imports, the setup and use are very similar
Get In Touch
We'd love to hear from you! Whether you have a question about our services, need a consultation, or just want to connect, our team is here to help. Reach out to us through the form, or contact us directly via social media.