Effective Java
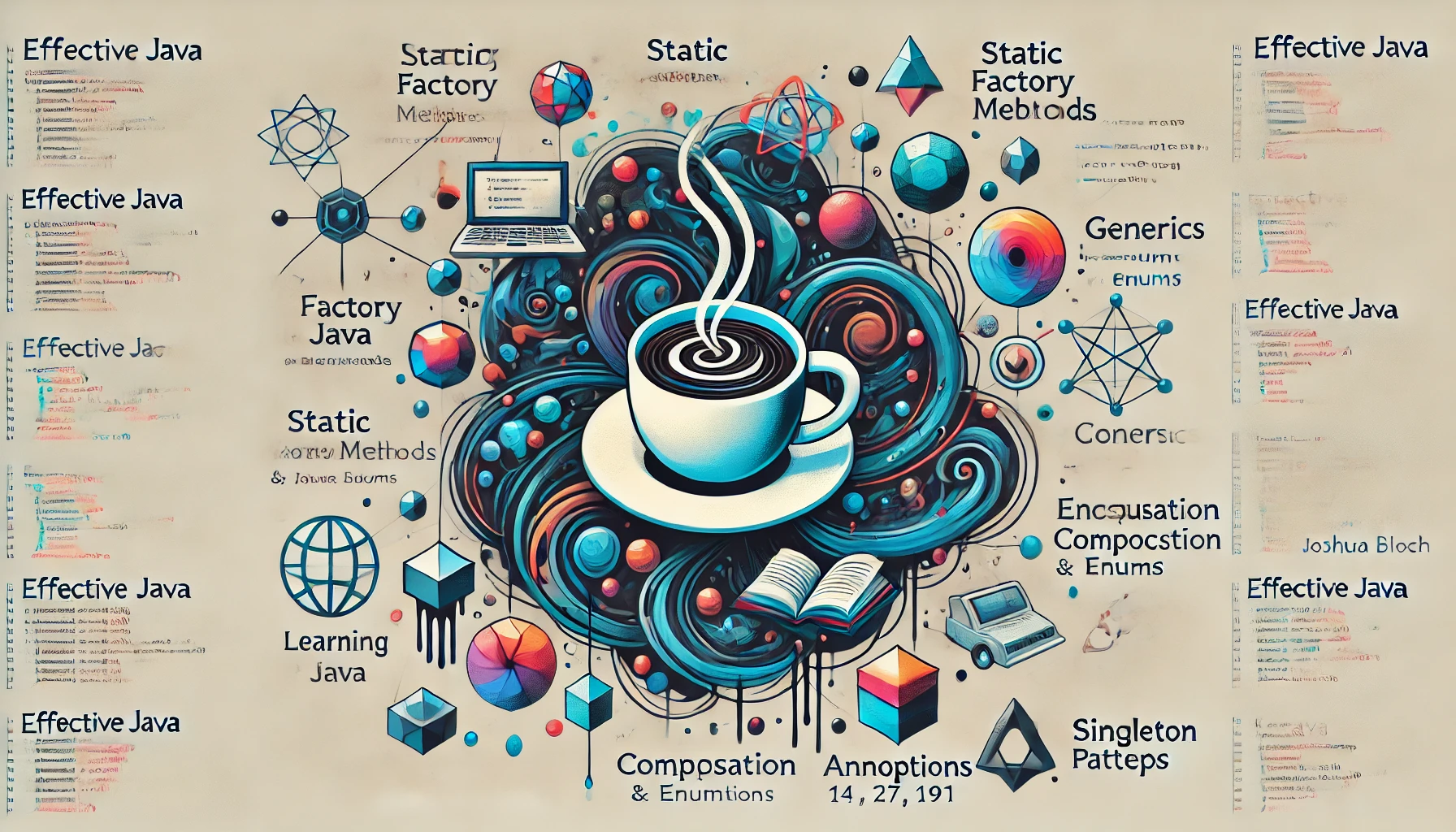
As an experienced software engineer who values extreme programming and software craftsmanship practices, I recently delved into Joshua Bloch's Effective Java. This book, now in its 2nd edition, is a staple for any Java developer looking to refine their skills and adopt best practices that lead to more robust, maintainable, and efficient code. While many of the concepts were familiar, Bloch's detailed explanations and the rationale behind each practice provided fresh insights and reinforced the importance of adhering to these guidelines.
Key Concepts from Effective Java:
Static Factory Methods:
- Descriptive Method Names: Static factory methods can be used to create objects with more descriptive (self-documenting) method names than a traditional new object constructor.
- Example:
Car cobaltCar = new Car("Cobalt");
vsCar cobaltCar = CarFactory.createCobalt();
- Benefit: By using the latter, changing what "defines" a cobalt can be abstracted outside of the Car class itself.
- Example:
- I rarely document my code with "what" comments and traditionally stick to "why" comments. The idea of using a general factory to help the code explain what is going on is a great concept.
- Descriptive Method Names: Static factory methods can be used to create objects with more descriptive (self-documenting) method names than a traditional new object constructor.
Minimize Accessibility and Mutability:
- Extreme Encapsulation: This practice involves taking encapsulation to an extreme level. By doing so, you limit the dependency of future extensions of the code and the client interface.
- Public Exposure: Only expose (public) what is absolutely needed, keeping the client interface manageable.
- Final Fields and Methods: Make fields and methods final to enforce good programming practices and limit extensions.
- Benefit: This reduces the likelihood of unexpected side effects and defects.
- This is another common practice, but the book talks about taking it to more of an extreme than what I traditionally considered best practice. By doing so you limit the dependency of future extensions of the code and the client interface.
- By only exposing (public) what is absolutely needed, the client interface is kept to a manageable set.
- By making fields and methods final, expansions of the code force good programming practices.
- Extreme Encapsulation: This practice involves taking encapsulation to an extreme level. By doing so, you limit the dependency of future extensions of the code and the client interface.
Prefer Composition Over Inheritance:
- Reduce Coupling: Composition reduces code dependency and coupling compared to inheritance.
- Fewer Touch Points: The fewer places a code change touches, the less likely unexpected side effects or defects will arise.
- I commonly do this within my code already, but the book enforced the idea that by doing so we reduce the code dependency and coupling.
- The fewer places a code change touches, the less likely unexpected side effects or defects will arise.
- Reduce Coupling: Composition reduces code dependency and coupling compared to inheritance.
Generics:
- Compile-Time Safety: Generics provide compile-time type safety that allows programmers to catch invalid types at compile time.
- Avoid ClassCastException: Generics help avoid
ClassCastException
at runtime by allowing the compiler to enforce type correctness. - Erasure: Java uses a concept called "erasure" to enforce compile-time constraints and ensure compatibility with older versions of Java.
- Years ago, generics were a foreign concept and a few older peer developers have resisted the concept. But, I hope by now we have all adopted the use of generics. I know .NET has byte code support for generics which provides runtime improvements, where Java uses a concept called "Erasure" that helps enforce compile-time enforcement within code to avoid
ClassCastException
at runtime.
Enums and Annotations:
- Enums: Use enums instead of
int
constants for better type safety and to represent fixed sets of constants. - Annotations: Use annotations to avoid boilerplate code and enhance readability. They provide a way to add metadata to your code, which can be processed by the compiler or at runtime.
- Enums: Use enums instead of
Effective Use of the Singleton Pattern:
- Singleton: Use the Singleton pattern to ensure a class has only one instance and provide a global point of access to it.
- Enum Type: The best way to implement a singleton in Java is by using an enum type.
- Singleton: Use the Singleton pattern to ensure a class has only one instance and provide a global point of access to it.
Critical Points and Unique Aspects of Effective Java:
Practical Advice: Bloch's book is filled with practical advice that stems from real-world experience, making it not just theoretical but highly applicable in day-to-day programming tasks.
Code Examples: The book includes numerous code examples that clearly illustrate the best practices being discussed. These examples help in understanding how to apply the concepts effectively.
Deep Dives: Bloch doesn't just list best practices; he provides deep dives into why these practices matter and the potential pitfalls of not following them. This level of detail helps in understanding the underlying principles of good software design.
Broad Coverage: The book covers a wide range of topics, from object creation and destruction to concurrency and serialization, providing a comprehensive guide to writing effective Java code.
Evolution of Java: The book also touches on the evolution of Java and how certain practices have emerged or changed with new versions of the language. This historical context is valuable for understanding the rationale behind certain practices.
Focus on Maintainability: A recurring theme in the book is writing code that is not just functional but maintainable. This aligns perfectly with the principles of software craftsmanship and extreme programming.
Get In Touch
We'd love to hear from you! Whether you have a question about our services, need a consultation, or just want to connect, our team is here to help. Reach out to us through the form, or contact us directly via social media.