The Hello World Kata
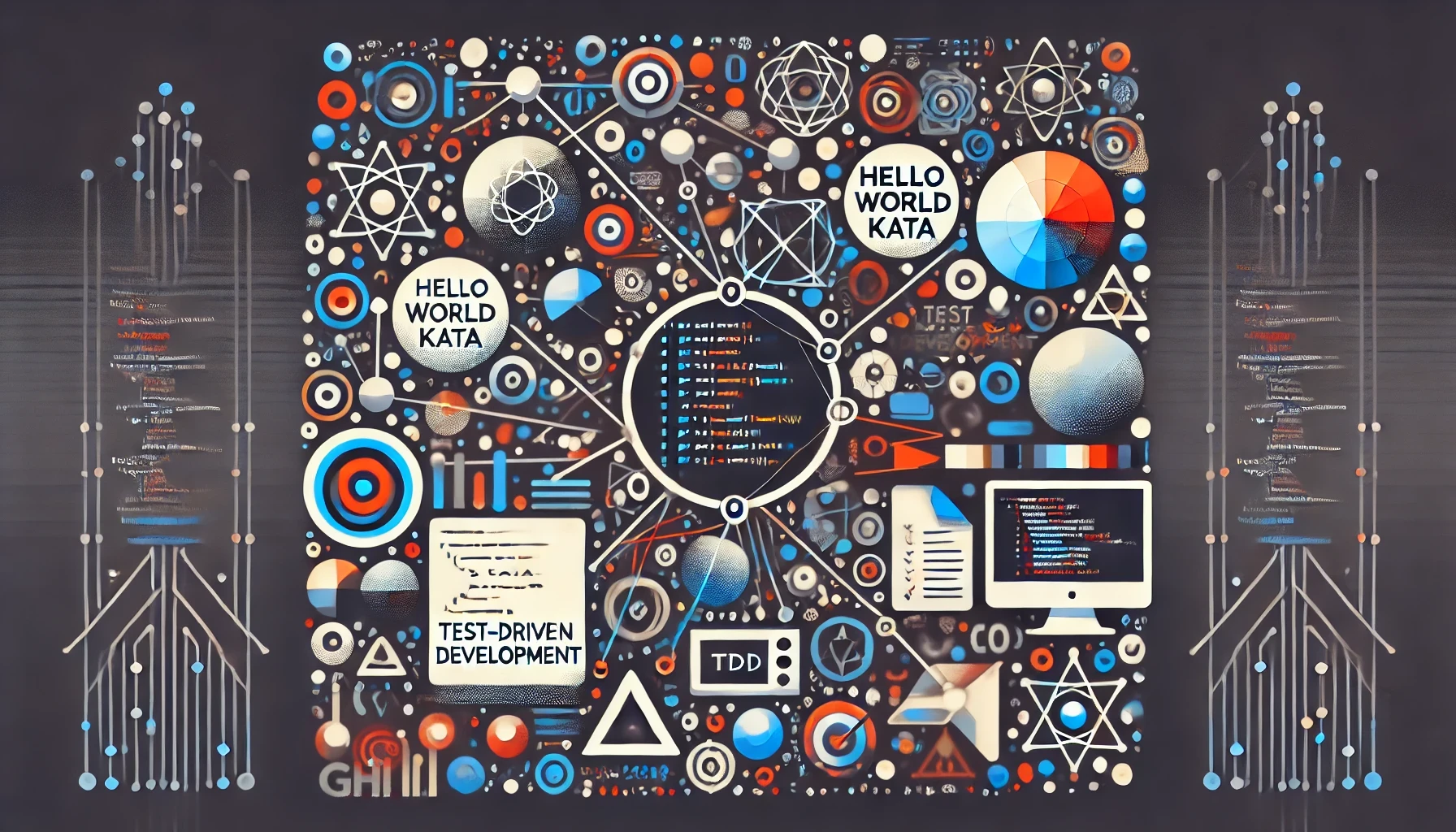
Hello World via Test Driven Development (TDD)
Pick a programming language (maybe the one you primarily use at work)... How hard/simple would it be to execute this kata?
I created a hello world python kata template: Hello World Kata
If you are already familiar with TDD, unit tests, and/or mocking, then this seems like an absurd exercise. Yet, this small program is enough to exercise each rule of TDD plus touch unit testing and mocking. These are the fundamental bases of TDD!
The intention of this article is to define, then walk through one solution to this kata.
Description
Expected Behavior
Create a program, which outputs to the console, 'hello world'.
Constraint
The three rules of TDD as Described by Uncle Bob
- You are not allowed to write any production code unless it is to make a failing unit test pass.
- You are not allowed to write any more of a unit test than is sufficient to fail; and compilation failures are failures.
- You are not allowed to write any more production code than is sufficient to pass the one failing unit test.
Solution (Python3)
Start by creating a test file hello_world_test.py. Assert nothing more than the file exits using an import statement. 1st + 2nd TDD Rule
import hello_world
test fails - ModuleNotFoundError: No module named 'hello_world'
Create hello_world.py as an empty file. 1st + 3rd TDD Rule
# no code, just a new file
test passes
The Python builtins print is called when the 'print' function is invoked. Update the test to mock this builtin... 2nd TDD Rule
from unittest.mock import patch
@patch('builtins.print')
def test_hello_world(mock_print):
import hello_world
mock_print.assert_called_with('hello world')
test fails : AssertionError: Expected call: print('hello world')
Write the feature code!!!
print('hello world')
test passes
- Profit! (Done)
Thoughts
Hello world is where a majority of software engineers begin at in any new language. In Python it is simply "print('hello world')".
Yet, one line of feature code is enough to expose the fundamentals of TDD!
Test Driven Development is a programming methodology that takes minutes to learn, but a career to master...
Now most programmers, when they first hear about this technique, think: “This is stupid!” “It’s going to slow me down, it’s a waste of time and effort, It will keep me from thinking, it will keep me from designing, it will just break my flow.” However, think about what would happen if you walked in a room full of people working this way. Pick any random person at any random time. A minute ago, all their code worked.
Get In Touch
We'd love to hear from you! Whether you have a question about our services, need a consultation, or just want to connect, our team is here to help. Reach out to us through the form, or contact us directly via social media.